I guess well find either way
no need to guess. ask chatGPT
you need to read it tho. might help with the spamming
TL;DR computers cannot understand code.
Why does the Halting Problem apply to computer programming, and how do we know it is true?
The Halting Problem applies to computer programming because it proves that there are limits to what we can predict about a program’s behavior using other programs or algorithms. The Halting Problem asks:
“Is there a general algorithm that can determine, for any given program and input, whether the program will eventually halt (finish running) or loop forever?” This question is deeply relevant to computer programming because programmers often want to know whether a piece of code will terminate or not—especially when trying to catch bugs or ensure a program is reliable.
We know the Halting Problem is true—and unsolvable in the general case—because
Alan Turing mathematically proved it in 1936. His proof showed that if such a universal halting algorithm existed, it would lead to a logical contradiction. He used a clever self-referential argument: he imagined a program that takes another program as input and does the opposite of what the halting algorithm says it would do—leading to a paradox. This means such a halting-checking program cannot exist.
Why this matters in programming:
- It proves that no tool can perfectly analyze every possible program to say whether it halts or not.
- It explains why automated program verification is inherently limited.
- It shows that some bugs (like infinite loops) might never be detected by analysis tools, no matter how smart they are.
So, the reason the Halting Problem applies to computer programming—and why we know it’s true—is because it’s a proven mathematical limitation on what computers can analyze about themselves and other programs.
-
What does the halting problem have to do with LLM code conversion from language to language?
The halting problem, a foundational concept in computability theory, plays an important role in understanding the
limits of what Large Language Models (LLMs) can do when converting code from one programming language to another. While LLMs can
appear to translate code successfully — for example, turning Python into C++ — they are fundamentally limited by the fact that
no algorithm can decide, in all cases, whether a program will halt (finish running) or run forever. This limitation directly applies to code translation tasks in subtle but significant ways.
Here's why:
- Preserving Behavior Requires Solving the Halting Problem (Which Is Impossible)
When converting code from language A to language B, ensuring that the new version behaves exactly the same includes ensuring it halts in the same situations. But due to the halting problem, there's no general way to determine whether arbitrary programs halt, let alone whether two translated versions halt in the same way. So LLMs can't guarantee behavior-preserving translations, especially for complex or unusual code.
- No Guarantee of Semantic Equivalence
Two programs written in different languages may look similar but behave differently if one halts and the other doesn’t. Since proving full semantic equivalence is undecidable in the general case (again, because of the halting problem), LLMs can only guess based on patterns learned from data — they don’t know whether the code is truly equivalent.
- Translation Quality Depends on Decidable Subsets
LLMs work well on common, well-behaved code where halting and correctness aren’t in question. But for anything nontrivial — recursive functions, dynamic input-driven loops, edge cases — they may silently introduce bugs or misbehavior during translation because they can’t reason about halting or correctness in a provable way.
TL;DR:
The halting problem has everything to do with LLM code conversion from language to language because it defines a hard limit on what can be known or proven about program behavior. Since LLMs cannot determine whether a piece of code will halt — and therefore whether its translated version behaves identically — they are fundamentally constrained in their ability to perform correct, reliable, and safe code translation at scale.
-
Why would LLMs find threaded programs harder to reason about?
LLMs (large language models) find threaded programs harder to reason about because
concurrency introduces complexity that’s not always explicitly written in the code. Threaded programs can behave differently depending on timing, race conditions, synchronization, and shared state—things that aren’t always obvious just by looking at the code.
Here’s why this is hard for LLMs:
- Non-determinism: In threaded programs, the same code can behave differently each time it runs because thread scheduling isn’t fixed. LLMs are trained on static patterns of code, so this unpredictability makes it harder to reason accurately.
- Hidden dependencies: Threads often share variables or resources. If one thread modifies a value while another reads it, the outcome depends on the exact timing—something LLMs can’t see from the code alone.
- Synchronization complexity: Constructs like locks, semaphores, and barriers add layers of logic that are subtle and easy to misuse. Understanding when and where locks are acquired or released requires precise tracking, which is challenging for models trained on language rather than executing code.
- Sparse training data: Threaded code is less common and more specialized in general programming datasets. That means LLMs may not have as much exposure to good examples or debugging patterns involving threads.
So,
LLMs struggle with threaded programs because the logic isn't always in the text—it’s in the invisible interplay between instructions over time.
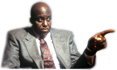